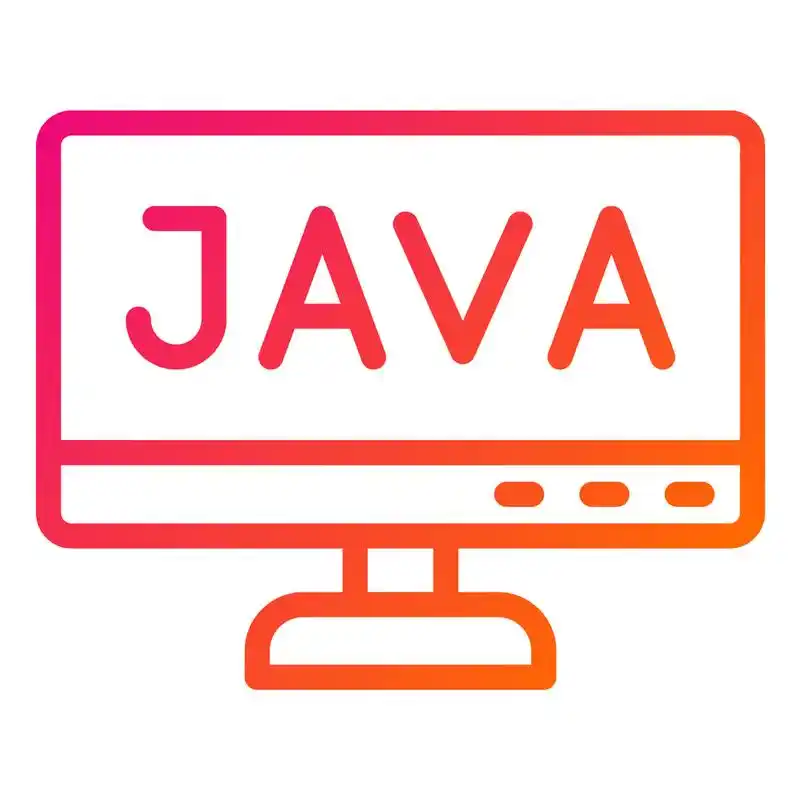
ImageButton 是 Android 中专门用于显示图片按钮的控件,它继承自 ImageView,但具有按钮的点击特性。下面我将全面介绍 ImageButton 的使用方法。
一、基本使用
1. XML 中声明 ImageButton
<ImageButton
android:id="@+id/imageButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_button_icon"
android:contentDescription="@string/button_desc"
android:scaleType="centerInside" />
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
2. 代码中设置图片
ImageButton imageButton = findViewById(R.id.imageButton);
// 设置图片资源
imageButton.setImageResource(R.drawable.ic_button_icon);
// 设置点击事件
imageButton.setOnClickListener(v -> {
// 处理点击事件
Toast.makeText(this, "ImageButton被点击", Toast.LENGTH_SHORT).show();
});
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
二、与普通 Button 的区别
特性 | ImageButton | Button |
---|---|---|
显示内容 | 只显示图片 | 可显示文字和/或图片 |
继承关系 | 继承自ImageView | 继承自TextView |
默认样式 | 无默认背景和点击效果 | 有系统默认的按钮样式 |
使用场景 | 纯图标按钮 | 文字按钮或图文混合按钮 |
三、高级用法
1. 不同状态下的图片显示
创建 selector 资源文件 (res/drawable/button_states.xml
):
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="true"
android:drawable="@drawable/ic_button_pressed" />
<item android:state_focused="true"
android:drawable="@drawable/ic_button_focused" />
<item android:drawable="@drawable/ic_button_normal" />
selector>
- 1
- 2
- 3
- 4
- 5
- 6
- 7
在布局中使用:
<ImageButton
android:id="@+id/imageButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/button_states"
android:background="@null" />
- 1
- 2
- 3
- 4
- 5
- 6
2. 添加点击水波纹效果
<ImageButton
android:id="@+id/imageButton"
android:layout_width="48dp"
android:layout_height="48dp"
android:src="@drawable/ic_button_icon"
android:background="?attr/selectableItemBackgroundBorderless" />
- 1
- 2
- 3
- 4
- 5
- 6
3. 圆形 ImageButton 实现
方法一:使用 CardView 包裹
<androidx.cardview.widget.CardView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:cardCornerRadius="24dp"
android:elevation="2dp">
<ImageButton
android:id="@+id/circleImageButton"
android:layout_width="48dp"
android:layout_height="48dp"
android:scaleType="centerCrop"
android:src="@drawable/profile_image" />
androidx.cardview.widget.CardView>
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
方法二:使用自定义背景
<ImageButton
android:id="@+id/circleImageButton"
android:layout_width="48dp"
android:layout_height="48dp"
android:background="@drawable/circle_bg"
android:src="@drawable/profile_image"
android:scaleType="centerCrop" />
- 1
- 2
- 3
- 4
- 5
- 6
- 7
res/drawable/circle_bg.xml
:
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="oval">
<solid android:color="#FF4081" />
shape>
- 1
- 2
- 3
- 4
四、实际应用示例
1. 实现一个拍照按钮
<ImageButton
android:id="@+id/cameraButton"
android:layout_width="64dp"
android:layout_height="64dp"
android:src="@drawable/ic_camera"
android:background="@drawable/circle_button_bg"
android:scaleType="centerInside"
android:elevation="4dp" />
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
circle_button_bg.xml
:
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="true">
<shape android:shape="oval">
<solid android:color="#B71C1C" />
<stroke android:width="2dp" android:color="#FFFFFF" />
shape>
item>
<item>
<shape android:shape="oval">
<solid android:color="#F44336" />
<stroke android:width="2dp" android:color="#FFFFFF" />
shape>
item>
selector>
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
2. 实现一个可切换的收藏按钮
ImageButton favoriteButton = findViewById(R.id.favoriteButton);
boolean isFavorite = false;
favoriteButton.setOnClickListener(v -> {
isFavorite = !isFavorite;
favoriteButton.setImageResource(isFavorite ?
R.drawable.ic_favorite_filled : R.drawable.ic_favorite_border);
// 添加动画效果
favoriteButton.animate()
.scaleX(1.2f)
.scaleY(1.2f)
.setDuration(100)
.withEndAction(() ->
favoriteButton.animate()
.scaleX(1f)
.scaleY(1f)
.setDuration(100)
.start())
.start();
});
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
五、性能优化与最佳实践
-
图片资源优化:
- 使用适当大小的图片资源
- 考虑使用 VectorDrawable 替代位图
- 为不同屏幕密度提供适配的资源
-
内存管理:
@Override protected void onDestroy() { super.onDestroy(); // 清除图片引用防止内存泄漏 imageButton.setImageDrawable(null); }
- 1
- 2
- 3
- 4
- 5
- 6
-
无障碍访问:
- 始终设置 contentDescription 属性
- 对功能性按钮添加更详细的描述
-
推荐使用 Material Design 图标:
<ImageButton android:id="@+id/materialButton" android:layout_width="48dp" android:layout_height="48dp" android:src="@drawable/ic_add_24dp" android:background="?attr/selectableItemBackgroundBorderless" android:tint="@color/primary" />
- 1
- 2
- 3
- 4
- 5
- 6
- 7
-
避免的常见错误:
- 忘记设置点击事件导致按钮无反应
- 图片过大导致OOM
- 未为不同状态提供视觉反馈
- 忽略无障碍访问需求
通过合理使用 ImageButton,可以创建直观、美观且功能完善的图标按钮,提升应用的用户体验。
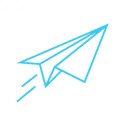
编程是艺术
微信公众号
文章主要分享软件开发和编程学习方面的知识


评论记录:
回复评论: